Creating Your Azure Terraform Backend
On Azure Blob Storage
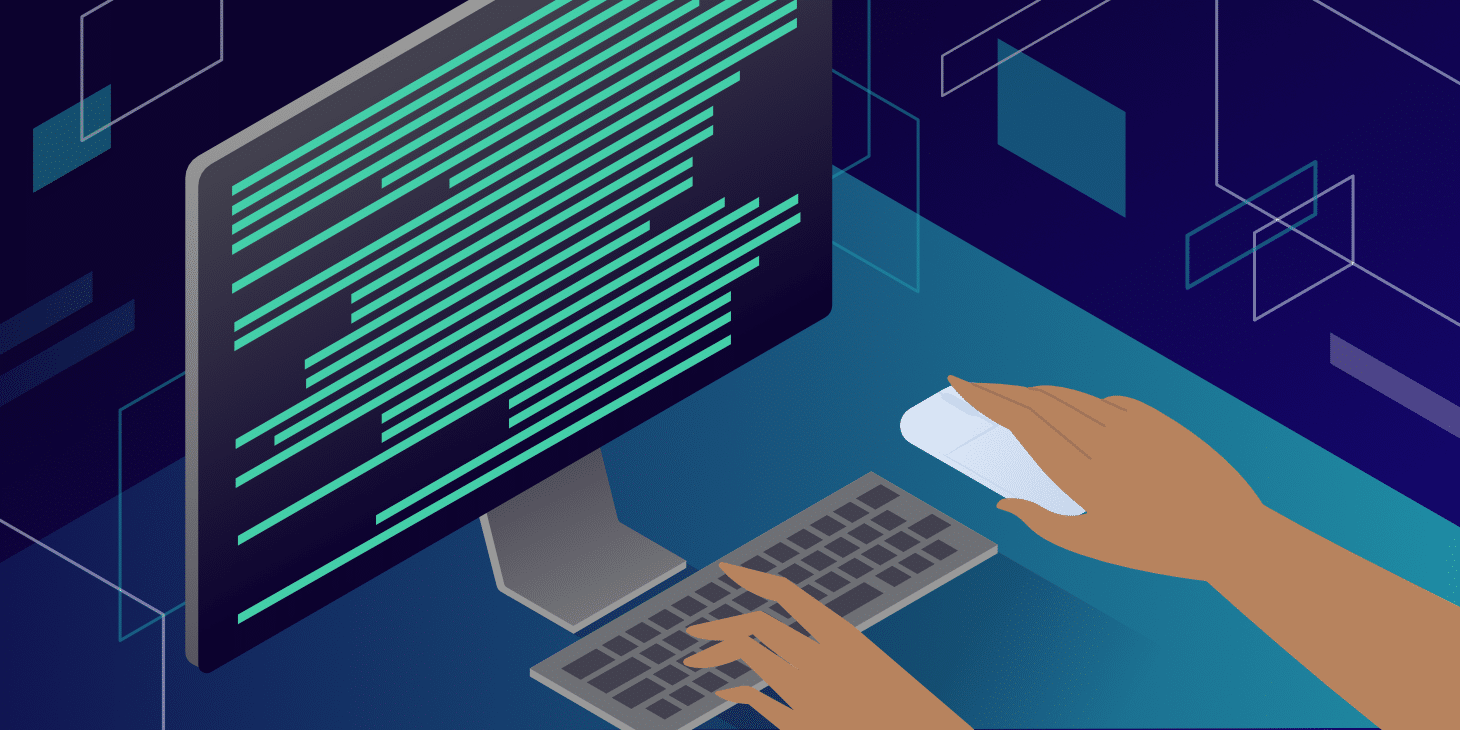
Managing Terraform state is crucial for the consistency and reliability of your infrastructure as code (IaC). Storing the Terraform state in a secure and accessible backend allows for collaboration and state locking, ensuring that multiple users or processes don't interfere with each other. Azure Blob Storage is an excellent choice for this purpose due to its scalability, security features, and seamless integration with Azure services.
In this blog post, we'll walk through creating an Azure Terraform backend using Azure Blob Storage. We'll cover the essential Terraform configurations, provide explanations, highlight customization options, and conclude with the full set of Terraform configuration files.
Terraform configuration files click here
Explanation of Terraform Configuration Files
provider.tf
The provider.tf file configures the Terraform provider, which is necessary to manage resources in Azure.
Code
#####################################################################
# provider.tf
#####################################################################
#####################################################################
# Provider Block 1
#####################################################################
# Azure Provider
provider "azurerm" {
features {}
}
version.tf
The version.tf file specifies the required Terraform version and the necessary providers for the project.
Code
#####################################################################
# version.tf
#####################################################################
#####################################################################
# Version Block
#####################################################################
# Provider Version
terraform {
required_version = ">=1.0"
required_providers {
azurerm = {
source = "hashicorp/azurerm"
version = "~>3.0"
}
random = {
source = "hashicorp/random"
version = "~>3.0"
}
}
}
random.tf
The random.tf file generates a unique identifier to ensure that the storage account name is globally unique.
Code
#####################################################################
# random.tf
#####################################################################
#####################################################################
# Resource Block 1
#####################################################################
# Generate random text for a unique storage account name
resource "random_id" "random_id" {
keepers = {
# Generate a new ID only when a new resource group is defined
resource_group = azurerm_resource_group.rg.name
}
byte_length = 6
}
local.tf
The local.tf file defines common tags that will be applied to all resources for consistency and easy management.
Code
#####################################################################
#local.tf
#####################################################################
#####################################################################
# Local Block 1
#####################################################################
# Create common tags using locals resource
locals {
common_tags = {
Environment = var.environment
Owner = var.owner
ProjectCode = var.projectcode
CostCenter = var.costcenter
}
}
variables.tf
The variables.tf file defines all the necessary variables used throughout the Terraform configuration.
Code
#####################################################################
# variables.tf
#####################################################################
#####################################################################
# Variables Block 1
#####################################################################
variable "prefix" {
type = string
default = "app"
description = "Prefix of the resource name"
}
variable "location" {
description = "Azure Location"
type = string
}
#####################################################################
# Variables Block 2
#####################################################################
variable "environment" {
description = "Environment Type - Prod/Stage/Dev"
type = string
}
variable "owner" {
description = "Environment Owner"
type = string
}
variable "projectcode" {
description = "Project Code"
type = string
}
variable "costcenter" {
description = "Cost Center"
type = string
}
#####################################################################
# Variables Block 3
#####################################################################
variable "account_tier" {
description = "Storage account tier"
type = string
}
variable "account_replication_type" {
description = "Storage account replication type"
type = string
}
variable "account_kind" {
description = "Storage account kind"
type = string
}
#####################################################################
# Variables Block 4
#####################################################################
variable "storage_container_name" {
description = "Storage Container Name"
type = string
}
variable "storage_container_type" {
description = "Storage Container Type"
type = string
}
terraform.tfvars
The terraform.tfvars file provides values for the variables defined in variables.tf.
Code
#####################################################################
# terraform.tfvars
#####################################################################
#####################################################################
# Variables Block 1
#####################################################################
# General
prefix = "sg1"
location = "East Us"
# Common Tags
environment = "Dev"
owner = "Roger Taylor"
projectcode = "0001"
costcenter = "c0001"
# Storage Account
account_tier = "Standard"
account_replication_type = "LRS"
account_kind = "StorageV2"
# Storage Account Container
storage_container_name = "tfstate"
storage_container_type = "private"
storageacct.tf
The storageacct.tf file creates the Azure Storage account and the container for the Terraform state files.
Code
#####################################################################
# storageacct.tf
#####################################################################
#####################################################################
# Resource Block 1
#####################################################################
# Create remote State Storage Account
resource "azurerm_storage_account" "stgacct" {
name = "${var.prefix}${random_id.random_id.hex}stgacct"
resource_group_name = azurerm_resource_group.rg.name
location = var.location
account_tier = var.account_tier
account_replication_type = var.account_replication_type
account_kind = var.account_kind
# Common Tags
tags = local.common_tags
depends_on = [azurerm_resource_group.rg]
}
#####################################################################
# Resource Block 2
#####################################################################
# Create remote State Storage Account Containter
resource "azurerm_storage_container" "stgcontainer" {
name = var.storage_container_name
storage_account_name = azurerm_storage_account.stgacct.name
container_access_type = var.storage_container_type
depends_on = [azurerm_storage_account.stgacct]
}
output.tf
The output.tf file provides outputs for important information about the created resources.
Code
#####################################################################
# output.tf
#####################################################################
#####################################################################
# Output Block 1
#####################################################################
# Resource Group Name
output "resourcegroupname" {
value = azurerm_resource_group.rg.name
}
# Resource Group Location
output "resourcegrouplocation" {
value = azurerm_resource_group.rg.location
}
#####################################################################
# Output Block 2
#####################################################################
# Storage Account Name
output "storageaccountname" {
value = azurerm_storage_account.stgacct.name
}
# Storage Account Access Tier
output "storageaccountaccesstier" {
value = azurerm_storage_account.stgacct.access_tier
}
# Storage Account Kind
output "storageaccountkind" {
value = azurerm_storage_account.stgacct.account_kind
}
Customization Options
- Resource Naming: Modify the prefix variable in terraform.tfvars to customize the resource naming convention.
- Location: Change the location variable in terraform.tfvars to deploy resources in different Azure regions.
- Tags: Customize the local.common_tags block in local.tf to include additional tags as needed.
- Storage Account Configuration: Adjust the account_tier, account_replication_type, and account_kind variables in terraform.tfvars to configure the storage account according to your requirements.
- Container Access Type: Modify the storage_container_type variable in terraform.tfvars to set the desired access level for the storage container (e.g., private, blob, or container).
Conclusion
Setting up an Azure Blob Storage backend for your Terraform state is a best practice for managing infrastructure as code. It provides benefits such as state locking, versioning, and secure storage, ensuring that your Terraform state is reliable and consistent.
The provided Terraform configuration files offer a comprehensive and customizable solution to create an Azure Blob Storage backend. By adjusting the variables and configurations, you can tailor the setup to fit your specific needs.
Happy Terraforming!
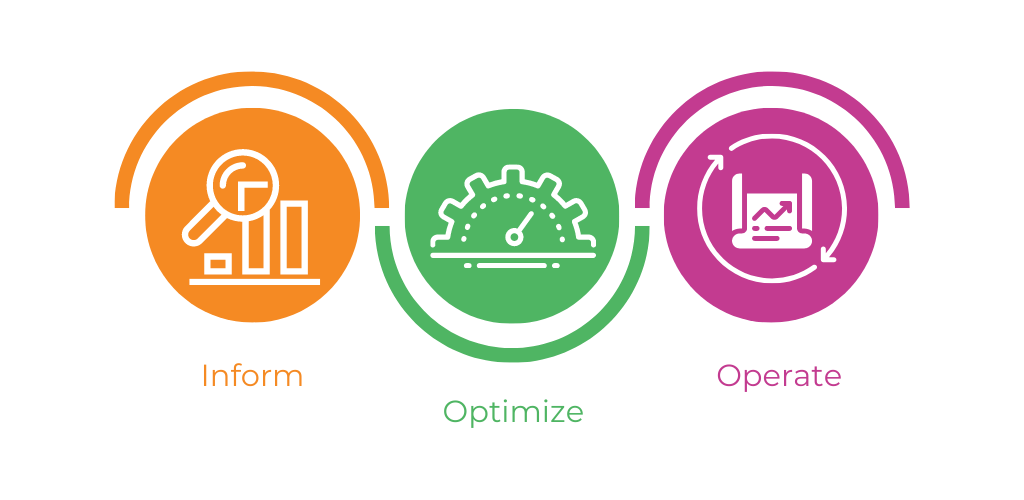
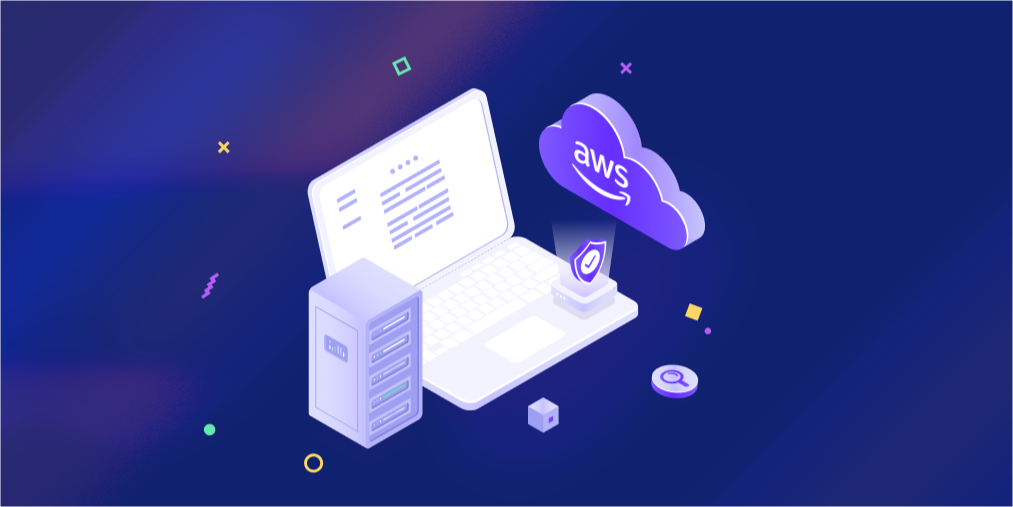
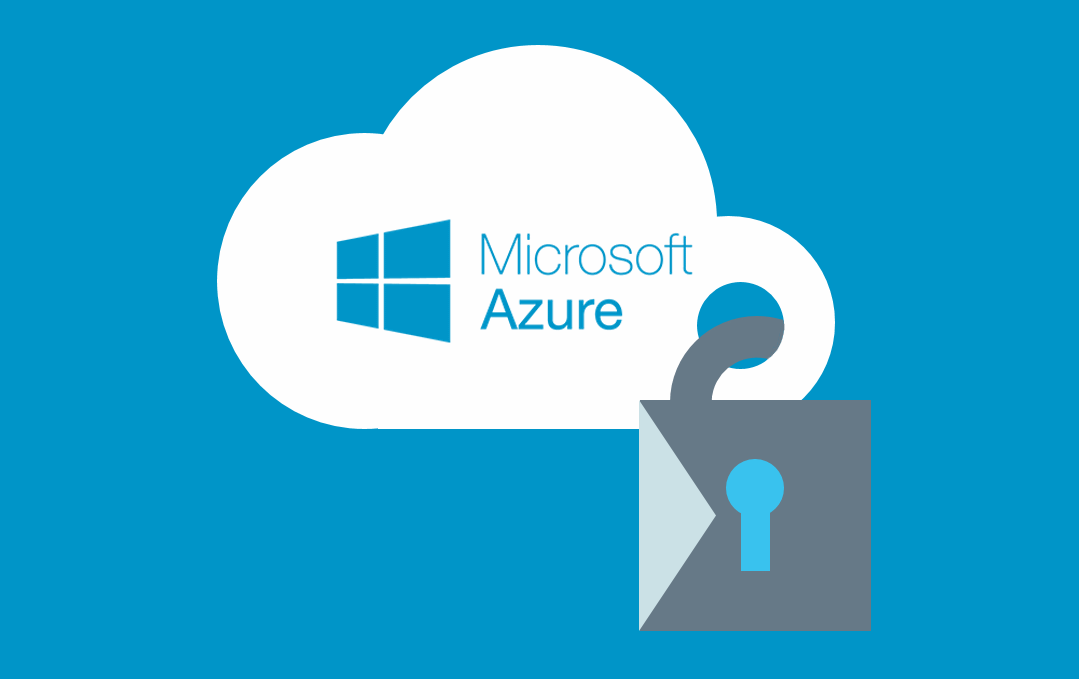
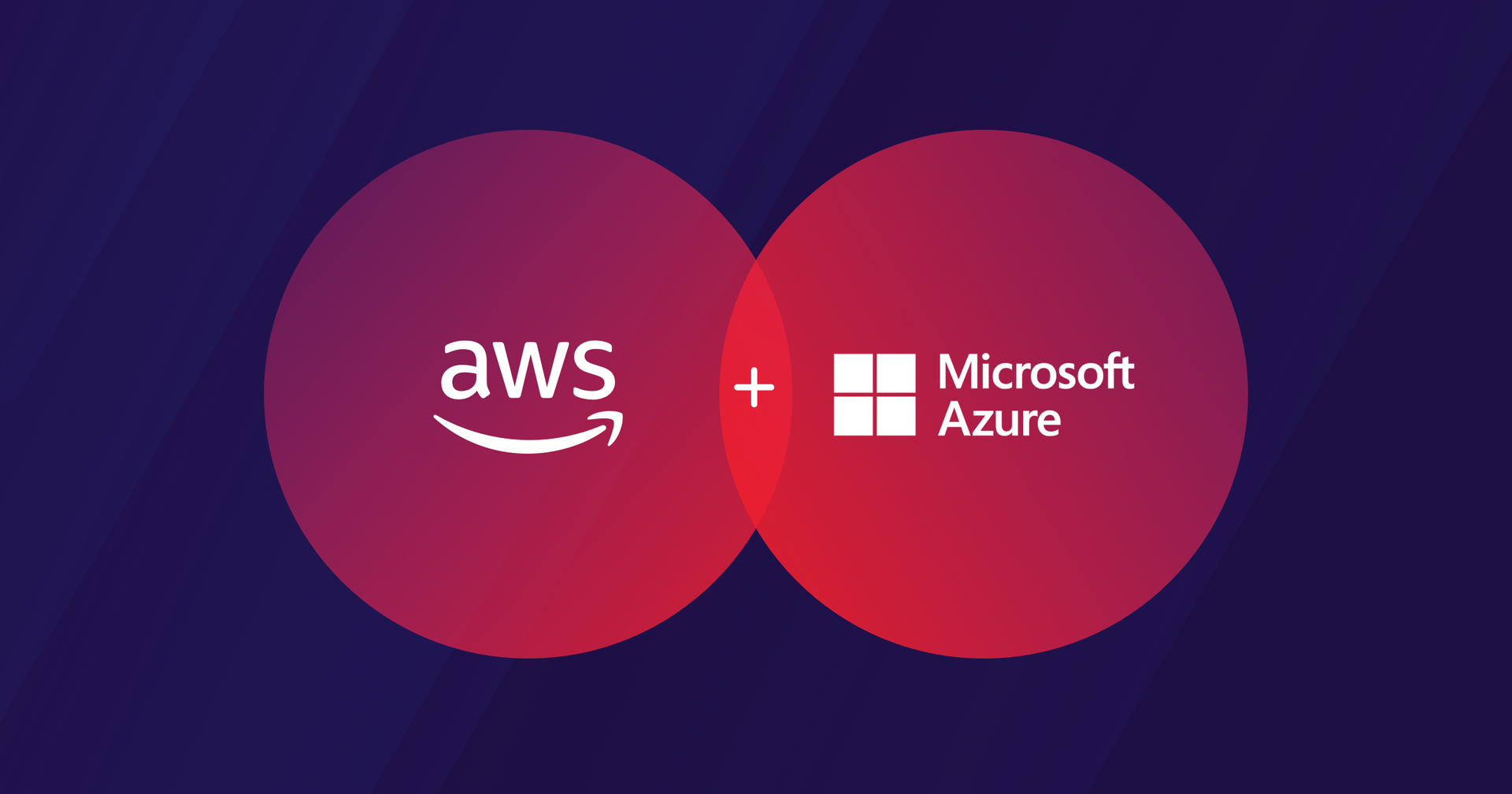
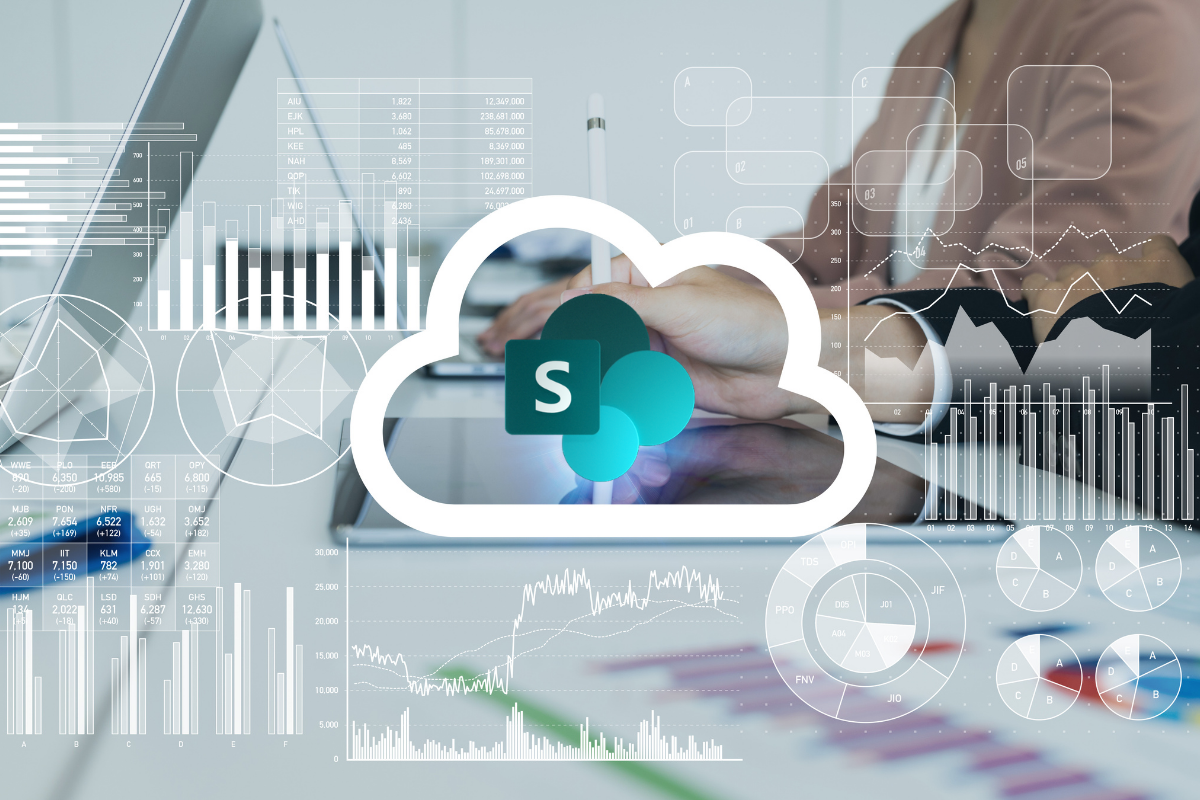